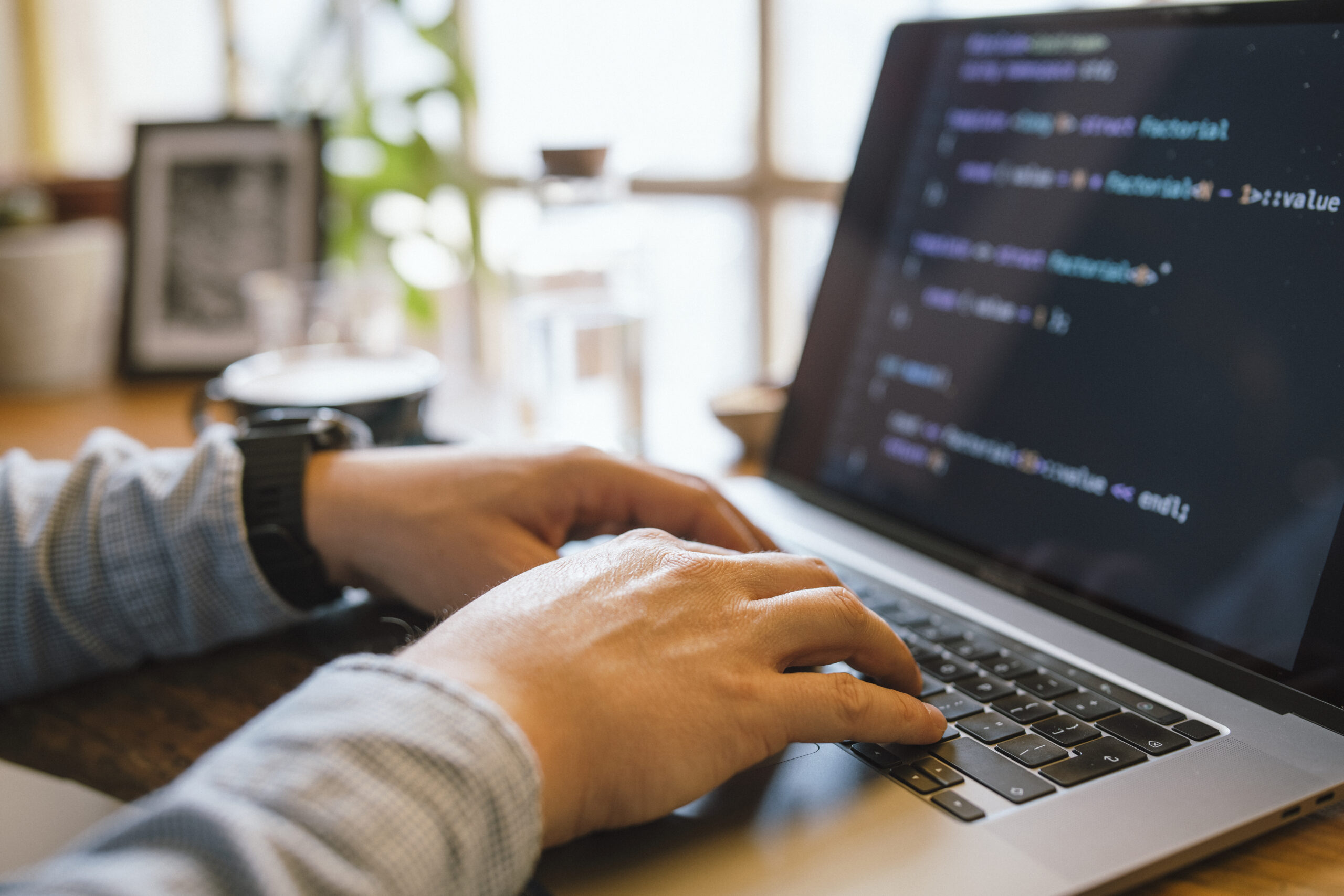
Debugging is One of the more important — still often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed below are a number of methods to help builders amount up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest strategies builders can elevate their debugging capabilities is by mastering the equipment they use every single day. Even though creating code is a single Section of enhancement, understanding the best way to communicate with it efficiently for the duration of execution is Similarly crucial. Modern day improvement environments arrive equipped with effective debugging capabilities — but lots of builders only scratch the surface area of what these tools can perform.
Consider, for example, an Built-in Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools permit you to set breakpoints, inspect the value of variables at runtime, phase by way of code line by line, as well as modify code within the fly. When applied accurately, they Allow you to notice precisely how your code behaves throughout execution, which can be priceless for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for front-conclude developers. They assist you to inspect the DOM, check community requests, view true-time overall performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI challenges into manageable duties.
For backend or process-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage over functioning processes and memory management. Discovering these equipment can have a steeper Studying curve but pays off when debugging overall performance challenges, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be relaxed with Variation Manage techniques like Git to be aware of code record, discover the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your applications means going past default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when difficulties occur, you’re not missing in the dead of night. The better you realize your tools, the more time you are able to invest solving the actual problem rather than fumbling via the process.
Reproduce the Problem
Probably the most critical — and infrequently missed — ways in helpful debugging is reproducing the condition. Right before leaping to the code or generating guesses, developers need to produce a reliable setting or circumstance where by the bug reliably seems. Devoid of reproducibility, repairing a bug will become a video game of opportunity, often bringing about squandered time and fragile code adjustments.
The first step in reproducing a problem is accumulating just as much context as you can. Request inquiries like: What steps resulted in the issue? Which environment was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you've got, the easier it will become to isolate the exact conditions below which the bug occurs.
As you’ve collected enough facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the situation but also avoid regressions Later on.
From time to time, the issue could be ecosystem-particular — it would materialize only on particular working devices, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a way of thinking. It requires patience, observation, along with a methodical strategy. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. That has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination likely fixes securely, and talk a lot more Obviously along with your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s where by builders prosper.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as annoying interruptions, developers really should understand to deal with error messages as immediate communications with the technique. They usually tell you exactly what took place, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information very carefully and in whole. A lot of developers, specially when below time pressure, look at the very first line and straight away start out producing assumptions. But further while in the error stack or logs may well lie the correct root lead to. Don’t just duplicate and paste mistake messages into search engines — read and recognize them 1st.
Break the mistake down into elements. Can it be a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line variety? What module or function activated it? These questions can tutorial your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, As well as in Those people instances, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and recent variations in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and assured developer.
Use Logging Correctly
Logging is Among the most impressive tools inside a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A superb logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts through progress, Data for basic activities (like effective start-ups), WARN for potential challenges that don’t split the appliance, ERROR for genuine troubles, and FATAL when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-considered-out logging method, you may reduce the time it will take to identify challenges, acquire further visibility into your purposes, and Increase the General maintainability and dependability within your code.
Think Like a Detective
Debugging is not simply a complex endeavor—it is a form of investigation. To properly establish and repair bugs, developers have to solution the process just like a detective resolving a secret. This state of mind aids stop working elaborate problems into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency difficulties. Identical to a detective surveys against the law scene, obtain just as much relevant information as you can with out jumping to conclusions. Use logs, check circumstances, and user experiences to piece with each other a clear picture of what’s happening.
Subsequent, type hypotheses. Inquire your self: What might be causing this behavior? click here Have any variations a short while ago been designed on the codebase? Has this situation transpired prior to less than very similar situation? The aim would be to narrow down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you nearer to the truth.
Pay near interest to compact specifics. Bugs often cover within the the very least anticipated sites—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue without the need of completely being familiar with it. Short-term fixes may perhaps conceal the actual problem, only for it to resurface afterwards.
Finally, retain notes on Everything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Write Exams
Composing checks is among the most effective approaches to increase your debugging competencies and overall advancement effectiveness. Assessments not simply enable capture bugs early but also serve as a safety net that gives you self-confidence when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug as it helps you to pinpoint accurately where and when a problem takes place.
Get started with device checks, which center on unique capabilities or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you promptly know the place to seem, drastically lowering time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following Beforehand remaining fastened.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These help ensure that several areas of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and less than what problems.
Creating exams also forces you to definitely Consider critically about your code. To check a attribute correctly, you'll need to be familiar with its inputs, predicted outputs, and edge instances. This volume of knowledge By natural means potential customers to better code composition and less bugs.
When debugging an issue, producing a failing test that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination go when the issue is settled. This technique makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Take Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy immediately after Option. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you're also close to the code for as well extended, cognitive fatigue sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging periods. Sitting before a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electrical power plus a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and more effective debugging Eventually.
In short, using breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, every one can teach you one thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start out by inquiring you a few key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code opinions, or logging? The solutions generally expose blind spots with your workflow or comprehension and allow you to Make more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see styles—recurring challenges or prevalent problems—that you can proactively stay clear of.
In workforce environments, sharing That which you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential areas of your improvement journey. In fact, a number of the best developers are usually not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques requires time, follow, and tolerance — however the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.